Drupal 8.x module banner using views
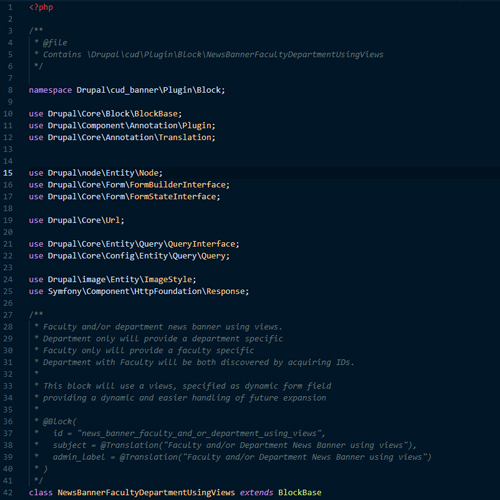
WordPress migration script that takes JSON values from a Drupal source. The scripts will automatically creates equivalent fields, slugs, taxonomies, slugs and path to ensure that the new migrated content is an exact replica from the source.
<?php /** * @file * Contains \Drupal\cud\Plugin\Block\NewsBannerFacultyDepartmentUsingViews */ namespace Drupal\cud_banner\Plugin\Block; use Drupal\Core\Block\BlockBase; use Drupal\Component\Annotation\Plugin; use Drupal\Core\Annotation\Translation; use Drupal\node\Entity\Node; use Drupal\Core\Form\FormBuilderInterface; use Drupal\Core\Form\FormStateInterface; use Drupal\Core\Url; use Drupal\Core\Entity\Query\QueryInterface; use Drupal\Core\Config\Entity\Query\Query; use Drupal\image\Entity\ImageStyle; use Symfony\Component\HttpFoundation\Response; /** * Faculty and/or department news banner using views. * Department only will provide a department specific * Faculty only will provide a faculty specific * Department with Faculty will be both discovered by acquiring IDs. * * This block will use a views, specified as dynamic form field * providing a dynamic and easier handling of future expansion * * @Block( * id = "news_banner_faculty_and_or_department_using_views", * subject = @Translation("Faculty and/or Department News Banner using views"), * admin_label = @Translation("Faculty and/or Department News Banner using views") * ) */ class NewsBannerFacultyDepartmentUsingViews extends BlockBase { /** * {@inheritdoc} */ public function blockForm($form, FormStateInterface $form_state) { $form = parent::blockForm($form, $form_state); $config = $this->getConfiguration(); $form['viewId'] = array( '#type' => 'textfield', '#title' => t('View Id to be rendered along with conditions'), '#default_value' => isset($config['viewId']) ? $config['viewId'] : '', ); $form['viewDisplayId'] = array( '#type' => 'textfield', '#title' => t('View Display Id. Inclusive only on the id of displays on the provided view (viewId field above).'), '#default_value' => isset($config['viewDisplayId']) ? $config['viewDisplayId'] : '', ); $form['departmentId'] = array( '#type' => 'number', '#title' => t('<strong>Department</strong> Node Id. If provided, force use and ignore context node id.'), '#default_value' => isset($config['departmentId']) ? $config['departmentId'] : 0, ); $form['facultyId'] = array( '#type' => 'number', '#title' => t('<strong>Faculty</strong> Node Id. If provided, force use and ignore context node id.'), '#default_value' => isset($config['facultyId']) ? $config['facultyId'] : 0, ); return $form; } /** * {@inheritdoc} */ public function blockSubmit($form, FormStateInterface $form_state) { $this->setConfigurationValue('viewId', $form_state->getValue('viewId')); $this->setConfigurationValue('viewDisplayId', $form_state->getValue('viewDisplayId')); $this->setConfigurationValue('facultyId', $form_state->getValue('facultyId')); $this->setConfigurationValue('departmentId', $form_state->getValue('departmentId')); } /** * {@inheritdoc} */ public function blockValidate($form, FormStateInterface $form_state) { $view_display_id = $form_state->getValue('viewDisplayId'); if (!is_numeric($view_display_id)) { $form_state->setErrorByName('viewDisplayId', t('Needs to be text')); } $view_id = $form_state->getValue('viewId'); if (!is_numeric($view_id)) { $form_state->setErrorByName('viewId', t('Needs to be text')); } $department_id = $form_state->getValue('departmentId'); if (!is_numeric($department_id)) { $form_state->setErrorByName('departmentId', t('Needs to be numeric')); } $faculty_id = $form_state->getValue('facultyId'); if (!is_numeric($faculty_id)) { $form_state->setErrorByName('facultyId', t('Needs to be numeric')); } } /** * Implements \Drupal\block\BlockBase::blockBuild(). */ public function build() { $config = $this->getConfiguration(); $view_id = $config['viewId']; $view_display_id = $config['viewDisplayId']; $arguments = array(); $faculty_id = $config['facultyId']; $department_id = $config['departmentId']; if (is_numeric($faculty_id) && $faculty_id > 0) { array_push($arguments, $faculty_id); } else { if ($node = \Drupal::service('current_route_match')->getParameter('node')) { if ($node->getType() == 'faculty') { array_push($arguments, $node->id()); } } } if (is_numeric($department_id) && $department_id > 0) { array_push($arguments, $department_id); } else { if ($node = \Drupal::service('current_route_match')->getParameter('node')) { if ($node->getType() == 'department') { if ($node->hasField('field_faculty') && $node->get('field_faculty')->count()) { $faculty = $node->get('field_faculty')->first()->getValue(); if ($faculty) { $faculty_id = $faculty['target_id']; array_push($arguments, $faculty_id); } } array_push($arguments, $node->id()); } } } $view = \Drupal\views\Views::getView($view_id); if ($view_display_id) { $view->setDisplay($view_display_id); if (count($arguments)) $view->setArguments($arguments); $view->execute(); return $view->render(); } return null; } }